This simple post explained the cookie handling including create,get and delete cookies by using javascript.In one of my ruby project I'm struggled with retained client side paging (Paging using javascript) on that time I'm using this client side cookies to over come that problem.I thing you may use this concept to client side paging,sorting,keep dropdown sclected index and other client side actions.
Code:
<script src="../cookies.js"></script>
Step 1: Check cookies is enabled
Before go to create cookie you have to check if the cookies are allowed.
<script type="text/javascript" language="javascript">
function isCookiesEnabled () {
if (testSessionCookie())
alert ("Session coookies are enabled")
else
alert ("Session coookies are not enabled");
}
</script>
Click to see if session cookies are allowed:
Step 2: Create cookie:
The next step you can give a name and value for your cookie.
<script type="text/javascript" language="javascript">
function setCookie(){
if (testSessionCookie()) {
var myName = document.getElementById('addCookiName').value;
var myValue = document.getElementById('addCookiValue').value;
createCookie (myName, myValue);
alert ("Session cookie created");
}
else
{
alert ("Sorry - session cookies are currently disabled.");
}
}
</script>
Demo:
Create cookie:
Cookie name:
Cookie value:
Step: 3: Get value from cookies by using its name
<script type="text/javascript" language="javascript">
function getCookie () {
var myCookieName = document.getElementById('getCookiName').value;
if (getCookieValue (myCookieName))
alert ('The value of the Cookie is "' + getCookieValue (myCookieName) + '"')
else
alert ("Cookie not found");
}
</script
Demo:
Get value from cookie by using it's name
Cookie name:
Step 4:
And finally you can delete value from cookie by using the following nethod
<script type="text/javascript" language="javascript">
function deleteCookies () {
var myCookieName = document.getElementById('deleteCookiName').value;
if(myCookieName != ""){
if (!getCookieValue (myCookieName))
alert ('Cookie does not exist')
else {
deleteCookie(myCookieName);
alert ("Cookie deleted successfully");
}
} else
{
alert ('Cookie does not exist')
}
}
</script>
Demo:
Delete value from cookie by using it's name
Cookie name:
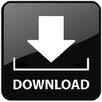